WPF 根據Word模版導出數據到Word
前臺.xaml文件:
<Window x:Class="Export.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="MainWindow" Height="350" Width="525">
<Grid>
<TextBox Height="23" HorizontalAlignment="Left" Margin="24,22,0,0" Name="textBox1" VerticalAlignment="Top" Width="322" />
<Button Content="Choose Stencil File" Height="23" HorizontalAlignment="Left" Margin="360,22,0,0" Name="button2" VerticalAlignment="Top" Width="131" Click="button2_Click" />
<Button Content="Export To Word" Height="44" HorizontalAlignment="Left" Margin="199,107,0,0" Name="ExportDoc" VerticalAlignment="Top" Width="100" Click="ExportDoc_Click" />
<Button Content="GetThumbnailsImage" Height="57" HorizontalAlignment="Left" Margin="160,228,0,0" Name="Thumbnails" VerticalAlignment="Top" Width="139" Click="Thumbnails_Click" />
</Grid>
</Window>
後臺.cs文件:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
using Microsoft.Office.Interop.Excel;
using System.Data;
using System.IO;
using System.Drawing.Imaging;
using System.Drawing;
namespace Export
{
/// <summary>
/// MainWindow.xaml 的交互邏輯
/// </summary>
public partial class MainWindow : System.Windows.Window
{
public MainWindow()
{
InitializeComponent();
}
private void button2_Click(object sender, RoutedEventArgs e)
{
var openFileDialog = new Microsoft.Win32.OpenFileDialog()
{
Filter = "Excel Files (*.xls,*.xlsx,*.xml)|*.xls;*.xlsx;*.xml"
};
var result = openFileDialog.ShowDialog();
if (result == true)
{
textBox1.Text = openFileDialog.FileName;
}
}
private System.Data.DataTable GetDt(){
//首先模擬建立將要導出的數據,這些數據都存於DataTable中
System.Data.DataTable dt = new System.Data.DataTable();
dt.Columns.Add("Name", typeof(string));
dt.Columns.Add("Gender", typeof(string));
dt.Columns.Add("Age", typeof(string));
dt.Columns.Add("Email", typeof(string));
dt.Columns.Add("Phone", typeof(string));
dt.Columns.Add("Adress", typeof(string));
DataRow row = dt.NewRow();
row["Name"] = "mzl";
row["Gender"] = "Male";
row["Age"] = "25";
row["Email"] = "[email protected]";
row["Phone"] = "12536885669";
row["Adress"] = "sfgagdgdfadg";
dt.Rows.Add(row);
row = dt.NewRow();
row["Name"] = "mzl2";
row["Gender"] = "Female";
row["Age"] = "23";
row["Email"] = "[email protected]";
row["Phone"] = "88654877";
row["Adress"] = "tyuutyj";
dt.Rows.Add(row);
return dt;
}
private void ExportDoc_Click(object sender, RoutedEventArgs e)
{
string filePath = textBox1.Text;
if (filePath == "")
{
MessageBox.Show("請選擇模版。");
return;
}
//獲取數據
System.Data.DataTable dt = GetDt();
System.IO.StreamReader sReader = new System.IO.StreamReader(filePath);
string strDocTemplate = sReader.ReadToEnd();
sReader.Close();
//將數據導入到word中
strDocTemplate = strDocTemplate.Replace("%Content%", dt.Rows[0]["Adress"].ToString());
strDocTemplate = strDocTemplate.Replace("%Name%", dt.Rows[0]["Name"].ToString());
strDocTemplate = strDocTemplate.Replace("%Age%", dt.Rows[0]["Age"].ToString());
strDocTemplate = strDocTemplate.Replace("%Gender%", dt.Rows[0]["Gender"].ToString());
strDocTemplate = strDocTemplate.Replace("%Phone%", dt.Rows[0]["Phone"].ToString());
//生成的壓縮圖片路徑
string strSourceFile = @"D:\Export\Template\a.jpg";
string strOutputFile = @"D:\Export\Template\b.jpg";
//壓縮圖片
GetThumbnailsImage(strSourceFile, 18, 3, @"D:\Export\Template\b.jpg");
//將圖像轉化爲base64的編碼
FileStream fs = new FileStream(strOutputFile, FileMode.Open);
byte[] byData = new byte[fs.Length];
fs.Read(byData, 0, byData.Length);
fs.Close();
string base64Photo = Convert.ToBase64String(byData);
//模版爲doc時(docx轉成doc的視爲docx模版),可以直接生成帶數據的標籤,然後替換字符串“%Photo%”
//本例使用WordTemplateDoc.xml模版
string strImageFormat = "<w:r><w:pict><v:shapetype id=\"_x0000_t75\" coordsize=\"21600,21600\" o:spt=\"75\" o:preferrelative=\"t\" path=\"m@4@5l@4@11@9@11@9@5xe\" filled=\"f\" stroked=\"f\"><v:stroke joinstyle=\"miter\"/><v:formulas><v:f eqn=\"if lineDrawn pixelLineWidth 0\"/><v:f eqn=\"sum @0 1 0\"/><v:f eqn=\"sum 0 0 @1\"/><v:f eqn=\"prod @2 1 2\"/><v:f eqn=\"prod @3 21600 pixelWidth\"/><v:f eqn=\"prod @3 21600 pixelHeight\"/><v:f eqn=\"sum @0 0 1\"/><v:f eqn=\"prod @6 1 2\"/><v:f eqn=\"prod @7 21600 pixelWidth\"/><v:f eqn=\"sum @8 21600 0\"/><v:f eqn=\"prod @7 21600 pixelHeight\"/><v:f eqn=\"sum @10 21600 0\"/></v:formulas><v:path o:extrusionok=\"f\" gradientshapeok=\"t\" o:connecttype=\"rect\"/><o:lock v:ext=\"edit\" aspectratio=\"t\"/></v:shapetype><w:binData w:name=\"wordml://03000001.png\" xml:space=\"preserve\">*ImageData*</w:binData><v:shape id=\"_x0000_i1025\" type=\"#_x0000_t75\" style=\"width:69.5pt;height:69.5pt\"><v:imagedata src=\"wordml://03000001.png\" o:title=\"nophoto\"/></v:shape></w:pict></w:r>";
string strImage = strImageFormat.Replace("*ImageData*", base64Photo);
strDocTemplate = strDocTemplate.Replace("%Photo%", strImage);
//模版爲docx時,圖片數據被放到xml文檔的結尾pkg標籤中了,可以用字符串“%PhotoTest%”替換掉已生成的圖片數據,然後直接傳入圖片數據的base64編碼進行替換,但是這時候模版無法再打開預覽了。
//本例使用WordTemplateDocx.xml模版
//strDocTemplate = strDocTemplate.Replace("%Photo%", base64Photo);
//生成word文件
string exportPath = "D:\\Export\\WordTest.doc";
if (File.Exists(exportPath))
{
File.Delete(exportPath);
}
FileStream fs1 = new FileStream(exportPath, FileMode.Create, FileAccess.Write);
StreamWriter sw = new StreamWriter(fs1, Encoding.UTF8);
sw.Write(strDocTemplate);
sw.Close();
fs1.Close();
MessageBox.Show("Export Complete!");
}
/// <summary>
/// 生成縮略圖
/// </summary>
/// <param name="sourceFile">原始圖片文件</param>
/// <param name="quality">質量壓縮比</param>
/// <param name="multiple">收縮倍數</param>
/// <param name="outputFile">輸出文件名</param>
/// <returns>成功返回true,失敗則返回false</returns>
public static bool GetThumbnailsImage(String sourceFile, long quality, int multiple, String outputFile)
{
try
{
long imageQuality = quality;
Bitmap sourceImage = new Bitmap(sourceFile);
ImageCodecInfo myImageCodecInfo = GetEncoderInfo("image/jpeg");
if (myImageCodecInfo == null)
{
MessageBox.Show("獲取圖片編碼失敗!");
}
System.Drawing.Imaging.Encoder myEncoder = System.Drawing.Imaging.Encoder.Quality;
EncoderParameters myEncoderParameters = new EncoderParameters(1);
EncoderParameter myEncoderParameter = new EncoderParameter(myEncoder, imageQuality);
myEncoderParameters.Param[0] = myEncoderParameter;
float xWidth = sourceImage.Width;
float yWidth = sourceImage.Height;
Bitmap newImage = new Bitmap((int)(xWidth / multiple), (int)(yWidth / multiple));
Graphics g = Graphics.FromImage(newImage);
g.DrawImage(sourceImage, 0, 0, xWidth / multiple, yWidth / multiple);
g.Dispose();
newImage.Save(outputFile, myImageCodecInfo, myEncoderParameters);
sourceImage.Dispose();
myEncoderParameters.Dispose();
myEncoderParameter.Dispose();
newImage.Dispose();
return true;
}
catch
{
return false;
}
}
/// <summary>
/// 獲取圖片編碼信息
/// </summary>
private static ImageCodecInfo GetEncoderInfo(String mimeType)
{
int j;
ImageCodecInfo[] encoders;
encoders = ImageCodecInfo.GetImageEncoders();
for (j = 0; j < encoders.Length; ++j)
{
if (encoders[j].MimeType == mimeType)
return encoders[j];
}
return null;
}
private void Thumbnails_Click(object sender, RoutedEventArgs e)
{
string pathPerc = @"D:\Export\Template\b.jpg";
string source = @"D:\Export\Template\a.jpg";
if (!File.Exists(pathPerc))
{
File.Create(pathPerc).Close();
}
else
{
File.Delete(pathPerc);
File.Create(pathPerc).Close();
}
GetThumbnailsImage(source, 18, 3, pathPerc);
MessageBox.Show("Complete!");
}
}
}
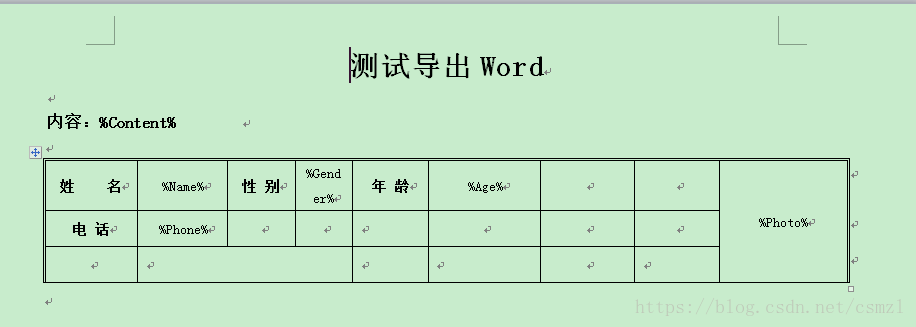
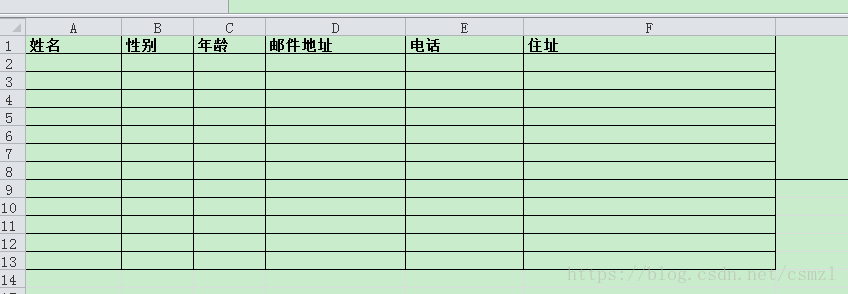
http://blog.csdn.net/sanjiawan/article/details/6818921
http://blog.csdn.net/szstephenzhou/article/details/38900345