Java常用類
文章目錄
一、String類
- java.lang.String類代表不可變的字符序列
- “xxxxxx”爲該類的一個對象
- String類的常見構造方法,具體可查看api文檔
- String類舉例:
public class TestString {
public static void main(String[] args) {
String s1 = "Hello";
String s2 = "world";
String s3 = "Hello";
System.out.println(s1 == s3);//true
s1 = new String("hello");
s2 = new String("hello");
System.out.println(s1 == s2);//false
System.out.println(s1.equals(s2));//true
char c[] = {'s','u','n',' ','j','a','v','a'};
String s4 = new String(c);
String s5 = new String(c,4,4);
System.out.println(s4);//sun java
System.out.println(s5);//java
}
}
二、String類小練習
編寫算法輸出一個字符串中的大寫英文字母數,小寫英文字母數以及非英文字符數
public class TestStringMethod {
public static void main(String[] args) {
int lcount = 0,ucount = 0,ncount = 0;
String s = "aaabbecHKILaaa$%^&Ab289HIQETRC";
for(int i = 0;i < s.length();i++){
char c = s.charAt(i);
if(c >= 'a' && c <= 'z'){
lcount ++;
}else if(c >= 'A' && c <= 'Z'){
ucount++;
}else {
ncount++;
}
}
System.out.println("小寫字母個數是 " + lcount + " 大寫字母的個數是 " + ucount
+ " 非英文的字符數是 " + ncount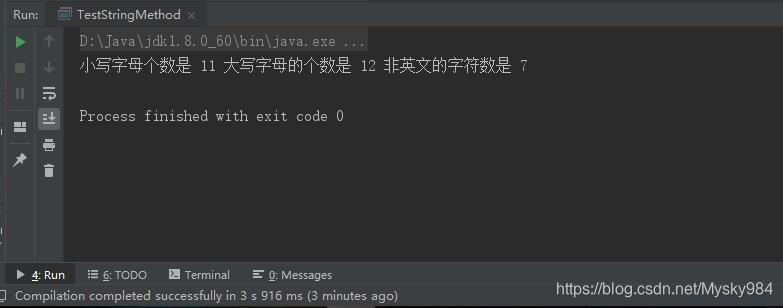);
}
}
輸出結果如下:
三、編寫一個方法,輸出在一個字符串中,指定字符串出現的次數。請編寫代碼實現。
public class AppearStringCount {
public static void main(String[] args) {
String s = "helloJavaGoodJavahahaJavaHiJava";
String sToFind = "Java";
int count = 0;
int index = -1;
while ((index = s.indexOf(sToFind)) != -1){
index = index + sToFind.length();
s = s.substring(index);
count++;
}
System.out.println(count);
}
}
四、基本數據類型包裝類
- 包裝類(如Integer,Double等)這些類封裝了一個相應的基本數據類型數值,併爲其提供了一系列操作,以java.lang.Integer類爲例,構造方法:
Integer(int value)
Integer(String s)
常用方法還有:Integer.parseInt()-轉換成int,基本數據類型轉換成一個對象,Long number = new Long(5)
即可,對象轉換成一個基本數據類型,number.longValue()
即可,其他的包裝類也有類似的方法。
- 包裝類常見方法。以下方法以java.lang.Integer爲例
public static final int MAX_VALUE //最大的int型數(2的31次方-1)
public static final int MIN_VALUE //最小的int型數(-2的31次方)
public long longValue() //返回封裝數據的long型值
public double doubleValue() //返回封裝數據的double型值
public int intValue() //返回封裝數據的int型值
public static int parseInt(String s) throws NumberFormatException //將字符串解析成int型數據,返回該數據
public static Integer valueOf(String s) throws NumberFormatException //返回Integer對象
五、小練習
編寫一個方法返回一個double類型的二維數組,數組中的元素通過解析字符串參數獲得。如字符串參數:“1,2;3,4,5;6,7,8”對應的數組爲:d[0,0] = 1.0,d[0,1] = 2.0,d[1,0] = 3.0,d[1,1] = 4.0,d[1,2] = 5.0,d[2,0] = 6.0,d[2,1] = 7.0,d[2,2] = 8.0。
public class ArrarParser {
public static void main(String[] args) {
double[][] d;
String s = "1,2;3,4,5;6,7,8";
String[] sFirst = s.split(";");
d = new double[sFirst.length][];
for(int i = 0;i < sFirst.length;i++){
String [] sSecond = sFirst[i].split(",");
d[i] = new double[sSecond.length];
for(int j = 0;j < sSecond.length;j++){
d[i][j] = Double.parseDouble(sSecond[j]);
}
}
/**
* 輸出二維數組
*/
for(int i = 0;i < d.length;i++){
for(int j = 0;j < d[i].length;j++){
System.out.print(d[i][j] + " ");
}
System.out.println();
}
}
}
六、Math類
java.lang.Math提供了一系列靜態方法用於科學計算,其方法的參數和返回值類型一般爲double類型,常用的方法如下:
abs//絕對值
acos,asin,atan,cos,sin,tan//三角函數
sqrt//平方根
pow(double a,double b)a的b次冪
log//自然對數
exp//爲底指數
max(double a,double b)//最大值
min(double a,double b)//最小值
random()//返回0.0到1.0之間的隨機數
long round(double a)//double型的數據a轉換爲long型(四捨五入)
toDegrees(double angrad)//弧度轉換爲角度
toRadians(double angoleg)//角度轉爲弧度
七、File類介紹
- java.io.File類代表系統文件名(路徑和文件名)
- File類的常見構造方法:
public File(String pathname)//以pathname爲路徑創建File對象,如果pathname是相對路徑,則默認的當前路徑在系統屬性user.dir中存儲
public File(String parent,String child)//以parent爲父路徑,child爲子路徑創建File對象
- File的靜態屬性String separator(分隔符)存儲了當前系統的路徑分隔符,此方法主要爲跨平臺的時候使用,其實路徑使用正斜槓"/"能達到同樣的效果。
- File類常用方法:
//1.通過File對象可以訪問文件的屬性
public boolean canRead()//是否可讀
public boolean canWrite()//是否可寫
public boolean exists()//文件是否存在
public boolean isDirectory()//是否是一個目錄
public boolean isFile()//是否是一個文件
public boolean isHidden()//是不是隱藏的
public long lastModefied()//上次修改的時間
public long length()//文件內容長度
public String getName()//獲得文件名字
public String getPath()/getAbsolutePath//獲的文件路徑/絕對路徑
//2.通過file對象創建空文件或目錄(在該對象所指的文件或目錄不存在的情況下)
public boolean createNewFile() throws IOException//創建文件
public boolean delete()//刪除文件
public boolean mkdir()//創建一個路徑(目錄)
public boolean mkdirs()//創建在路徑中的一系列目錄
八、編寫一個程序,在命令行中以樹狀結構展現特定的文件夾及其子文件和子文件夾
import java.io.File;
public class FileList {
public static void main(String[] args) {
File f = new File("D:/A");
System.out.println(f.getName());
tree(f,1);
}
/**
* 遞歸方式實現
* @param f 文件
* @param level 添加目錄層次
*/
private static void tree(File f,int level){
String preStr = " ";
for(int i = 0;i < level;i++){
preStr += " ";
}
File[] childs = f.listFiles();
for(int i = 0;i<childs.length;i++){
System.out.println(preStr + childs[i].getName());
if(childs[i].isDirectory()){
tree(childs[i],level + 1);
}
}
}
}
輸出結果:
九、java.lang.Enum枚舉類型
- 只能夠取特定值中的一個
- 使用enum關鍵字
- 是java.lang.Enum類型
- 使用枚舉類型目的就是限制某些值,使其能夠在編譯過程中就能夠限制,而免去了在運行時的判斷,能夠精簡代碼,防止錯誤的發生。
- 小例子如下:
public class TestEnum {
/**
* 定義一個enum類型
*/
public enum MyCloor {
red,
green,
blue
}
public static void main(String[] args) {
MyCloor m = MyCloor.red;
switch (m) {
case red:
System.out.println("red");
break;
case green:
System.out.println("green");
break;
case blue:
System.out.println("blue");
break;
default:
break;
}
}
}