@[TOC](回望Spring旅程(持續更新))
# 第一章節 Spring之旅
## 1.1 爲什麼會創建Spring
Spring在誕生之初,主要目的就是爲了替代更加重量級的企業級Java技術,提供更加輕量級和簡單的編程模型。增強老式Java對象的功能,讓他具備了之前只有EJB和其他企業級Java規範纔有的功能。
## 1.2 簡化Java開發
爲了降低Java開發的複雜性,Spring採取了以下4種關鍵性的策略:
- 基於POJO的輕量級和最小侵入式編程
- 通過依賴注入和麪向接口實現了松耦合
- 基於切面和慣例實現聲明式編程
- 通過切面和模板減少樣板式代碼
### 1.2.1 爲什麼說是基於POJO的輕量級和最小侵入式編程呢?
如何理解這句話?
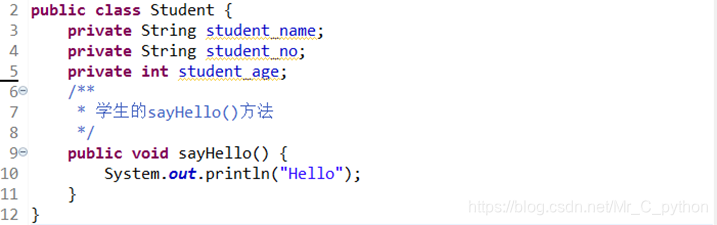
如上,即便這個Student對象使用了Spring,但是如果放到一個非Spring的應用中,依舊是可以當作一個POJO對象(但是可能註解會報錯,這也是最壞的情況,但依舊不影響他是一個POJO)。
我們都知道Spring的DI是有兩種方法,待會會講到,使用註解後放到另一個沒有Spring的程序中,Spring的註解會報錯沒有導包,但如果是xml注入,完全沒有影響,因此這裏也說是最壞的情況。
### 1.2.2 通過依賴注入和麪向接口實現松耦合是什麼意思?
#### 1.2.2.1 依賴注入的兩種實現方式
##### 基於xml的依賴注入:
1. Student學生對象:
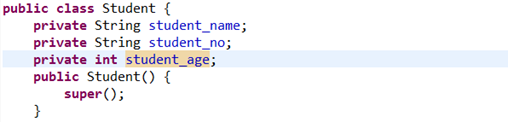
2. 進行依賴注入:applicationContext.xml文件(Spring IOC上下文文件,也叫做IOC容器)
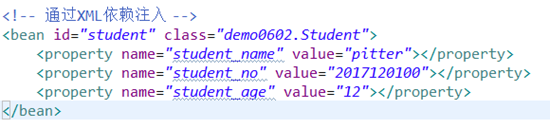
3. 測試程序:

4. 結果:(sayHello()是Student的一個方法)
> Hello
##### 基於註解的依賴注入:
1. Student學生對象
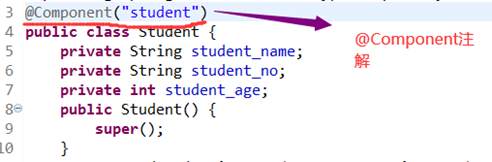
3. 進行依賴注入:

4. 測試:

#### 1.2.2.2 什麼是松耦合?
##### 傳統的對象實例化
```java
Student student = new Student();
```
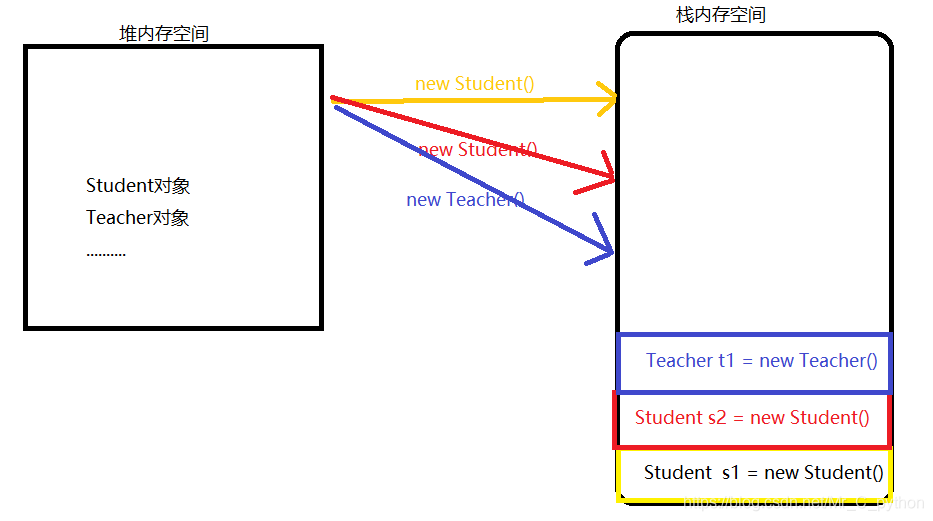
根據圖解,可以知道,在我們創建一個類之後,相應的會在堆內存中的某一個地址,存放這個對象。當我們實例化這個對象時,會從堆內存中把指向這個對象的地址壓入棧內存之中。
如果按照傳統的```new```實例化對象,如果只是一兩個實例那還好,如果某一個大型的項目,豈不是要```new```它個上萬次?不佔內存嗎?不會影響性能嗎?有槓精就要說了,怕啥,內存我有的是。
但是你每次都去```new```,項目模塊之間的耦合性會不會因此受到影響?爲了解決松耦合的問題,就出現了```單例設計模式```
##### 單例設計模式
所謂單例模式,就是保證類在內存中只有一個對象,分爲餓漢式、懶漢式
- [x] 餓漢式單例設計模式
```java
public class Fruit {
private String name;
private double price;
//本類實例化自己(private)
private static Fruit fruit = new Fruit();
//私有的構造,爲了不讓外部實例化
private Fruit(String name, double price) {
super();
this.name = name;
this.price = price;
}
//私有的構造,爲了不讓外部實例化
private Fruit() {
super();
}
//但是我們要設置一個方法讓外部可以調用獲取對象
public static Fruit getFruit() {
return fruit;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
@Override
public String toString() {
return "Fruit [name=" + name + ", price=" + price + "]";
}
}
```
```java
public static void main(String[] args) {
//獲取實例化對象
Fruit fruit = Fruit.getFruit();
}
```
- [x] 懶漢式單例設計模式
```java
public class Fruit {
private String name;
private double price;
//本類實例化自己(private)
private static Fruit fruit;
//私有的構造,爲了不讓外部實例化
private Fruit(String name, double price) {
super();
this.name = name;
this.price = price;
}
//私有的構造,爲了不讓外部實例化
private Fruit() {
super();
}
//但是我們要設置一個方法讓外部可以調用獲取對象
public static Fruit getFruit() {
if(fruit==null) {
fruit = new Fruit();
}
return fruit;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
@Override
public String toString() {
return "Fruit [name=" + name + ", price=" + price + "]";
}
}
```
```java
public static void main(String[] args) {
Fruit fruit1 = Fruit.getFruit();
Fruit fruit2 = Fruit.getFruit();
System.out.println(fruit1==fruit2); //輸出結果:true
}
```
> <font color="blue">餓漢式:簡單來說就是空間換時間,因爲上來就實例化一個對象,佔用了內存,(也不管你用還是不用)
懶漢式:簡單的來說就是時間換空間,與餓漢式正好相反</font>
##### 工廠設計模式
再後來,設計了工廠設計模式。工廠模式是我們最常用的實例化對象模式了,是用工廠方法代替new操作的一種模式。著名的Jive論壇 ,就大量使用了工廠模式,工廠模式在Java程序系統可以說是隨處可見。因爲工廠模式就相當於創建實例對象的new,我們經常要根據類Class生成實例對象,如A a=new A() 工廠模式也是用來創建實例對象的,所以以後new時就要多個心眼,是否可以考慮使用工廠模式,雖然這樣做,可能多做一些工作,但會給你係統帶來更大的可擴展性和儘量少的修改量。
```java
public class Banana {
private String name;
public Banana(String name) {
super();
this.name = name;
}
public Banana() {
super();
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@Override
public String toString() {
return "Apple [name=" + name + "]";
}
}
```
```java
public class Apple {
private String name;
public Apple(String name) {
super();
this.name = name;
}
public Apple() {
super();
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@Override
public String toString() {
return "Apple [name=" + name + "]";
}
}
```
```java
public class Orange {
private String name;
public Orange(String name) {
super();
this.name = name;
}
public Orange() {
super();
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@Override
public String toString() {
return "Apple [name=" + name + "]";
}
}
```
```java
public class FruitFactory {
//工廠方法
public Object getObject(String name) {
if(name=="apple") {
return new Apple();
}
if(name=="banana") {
return new Banana();
}
if(name=="orange") {
return new Orange();
}
return null;
}
}
```
```java
public static void main(String[] args) {
//從工廠獲取Apple對象實例
Apple apple = (Apple) new FruitFactory().getObject("apple");
}
```
##### Spring控制反轉
我們可以看見單例設計模式下,對象只會被實例化一次,但是我們會發現我們的對象就變得有些許不像是POJO對象了。爲了更好的解決松耦合問題,我們引入了```控制反轉(依賴注入&依賴查找)```
<font color="blue" size="3">1.什麼是控制反轉?</font>
<font color="grey" size="2">Class A中用到了Class B的對象b,一般情況下,需要在A的代碼中顯式的new一個B的對象。
採用依賴注入技術之後,A的代碼只需要定義一個私有的B對象,不需要直接new來獲得這個對象,而是通過相關的容器控制程序來將B對象在外部new出來並注入到A類裏的引用中。而具體獲取的方法、對象被獲取時的狀態由配置文件(如XML)來指定。
可以把IoC模式看作工廠模式的昇華,把IoC容器看作是一個大工廠,只不過這個大工廠裏要生成的對象都是在XML文件中給出定義的。利用Java 的“反射”編程,根據XML中給出的類定義生成相應的對象。從實現來看,以前在工廠模式裏寫死了的對象,IoC模式改爲配置XML文件,這就把工廠和要生成的對象兩者隔離,極大提高了靈活性和可維護性。
IoC中最基本的Java技術就是“反射”編程。通俗的說,反射就是根據給出的類名(字符串)來生成對象。這種編程方式可以讓應用在運行時才動態決定生成哪一種對象。反射的應用是很廣泛的,像Hibernate、Spring中都是用“反射”做爲最基本的技術手段。
在過去,反射編程方式相對於正常的對象生成方式要慢10幾倍,這也許也是當時爲什麼反射技術沒有普遍應用開來的原因。但經SUN改良優化後,反射方式生成對象和通常對象生成方式,速度已經相差不大了(但依然有一倍以上的差距)。
</font>
<font color="blue" size="3">2、SpringIOC容器形式</font>
<font color="grey" size="2">IoC是一個很大的概念,可以用不同的方式實現。其主要形式有兩種:
**依賴查找:** 容器提供回調接口和上下文條件給組件。EJB和Apache Avalon 都使用這種方式。這樣一來,組件就必須使用容器提供的API來查找資源和協作對象,僅有的控制反轉只體現在那些回調方法上(也就是上面所說的 類型1):容器將調用這些回調方法,從而讓應用代碼獲得相關資源。
**依賴注入:** 組件不做定位查詢,只提供普通的Java方法讓容器去決定依賴關係。容器全權負責的組件的裝配,它會把符合依賴關係的對象通過JavaBean屬性或者構造函數傳遞給需要的對象。通過JavaBean屬性注射依賴關係的做法稱爲設值方法注入(Setter Injection);將依賴關係作爲構造函數參數傳入的做法稱爲構造器注入(Constructor Injection)
</font>
### 1.2.3 依賴注入
==Student類==
```java
package day0610.pojo;
public class Student {
private String sname,sno;
public Student() {
super();
}
public Student(String sname, String sno) {
super();
this.sname = sname;
this.sno = sno;
}
public String getSname() {
return sname;
}
public void setSname(String sname) {
this.sname = sname;
}
public String getSno() {
return sno;
}
public void setSno(String sno) {
this.sno = sno;
}
@Override
public String toString() {
return "Student [sname=" + sname + ", sno=" + sno + "]";
}
}
```
#### 1.2.3.1 通過Setter進行依賴注入
```xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:p="http://www.springframework.org/schema/p"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.3.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.3.xsd">
<!-- this is bean for student -->
<bean id="student" class="day0610.pojo.Student">
<property name="sname" value="tom"/>
<property name="sno" value="110001"/>
</bean>
</beans>
```
```java
package day0610.main;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import day0610.pojo.Student;
public class Test {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
Student student = (Student)context.getBean("student");
System.out.println(student);
}
}
```
輸出結果:
```
Student [sname=tom, sno=110001]
```
現在我們將我們POJO類的```setter()```方法拿掉,再輸出
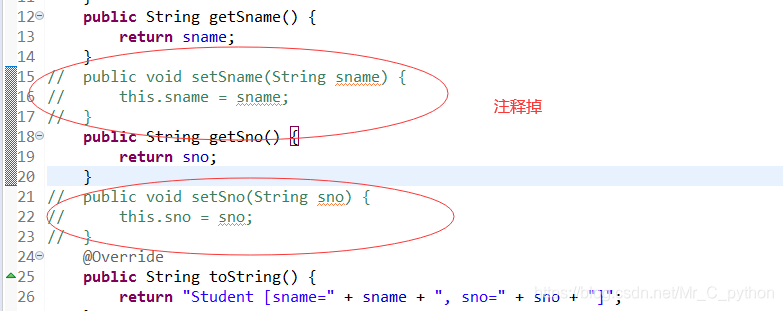
得到報錯結果:
```
六月 10, 2020 6:07:56 下午 org.springframework.context.support.AbstractApplicationContext refresh
警告: Exception encountered during context initialization - cancelling refresh attempt: org.springframework.beans.factory.BeanCreationException: Error creating bean with name 'student' defined in class path resource [applicationContext.xml]: Error setting property values; nested exception is org.springframework.beans.NotWritablePropertyException: Invalid property 'sname' of bean class [day0610.pojo.Student]: Bean property 'sname' is not writable or has an invalid setter method. Does the parameter type of the setter match the return type of the getter?
Exception in thread "main" org.springframework.beans.factory.BeanCreationException: Error creating bean with name 'student' defined in class path resource [applicationContext.xml]: Error setting property values; nested exception is org.springframework.beans.NotWritablePropertyException: Invalid property 'sname' of bean class [day0610.pojo.Student]: Bean property 'sname' is not writable or has an invalid setter method. Does the parameter type of the setter match the return type of the getter?
at org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.applyPropertyValues(AbstractAutowireCapableBeanFactory.java:1743)
at org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.populateBean(AbstractAutowireCapableBeanFactory.java:1451)
at org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.doCreateBean(AbstractAutowireCapableBeanFactory.java:594)
at org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.createBean(AbstractAutowireCapableBeanFactory.java:517)
at org.springframework.beans.factory.support.AbstractBeanFactory.lambda$doGetBean$0(AbstractBeanFactory.java:323)
at org.springframework.beans.factory.support.DefaultSingletonBeanRegistry.getSingleton(DefaultSingletonBeanRegistry.java:222)
at org.springframework.beans.factory.support.AbstractBeanFactory.doGetBean(AbstractBeanFactory.java:321)
at org.springframework.beans.factory.support.AbstractBeanFactory.getBean(AbstractBeanFactory.java:202)
at org.springframework.beans.factory.support.DefaultListableBeanFactory.preInstantiateSingletons(DefaultListableBeanFactory.java:879)
at org.springframework.context.support.AbstractApplicationContext.finishBeanFactoryInitialization(AbstractApplicationContext.java:878)
at org.springframework.context.support.AbstractApplicationContext.refresh(AbstractApplicationContext.java:550)
at org.springframework.context.support.ClassPathXmlApplicationContext.<init>(ClassPathXmlApplicationContext.java:144)
at org.springframework.context.support.ClassPathXmlApplicationContext.<init>(ClassPathXmlApplicationContext.java:85)
at day0610.main.Test.main(Test.java:7)
Caused by: org.springframework.beans.NotWritablePropertyException: Invalid property 'sname' of bean class [day0610.pojo.Student]: Bean property 'sname' is not writable or has an invalid setter method. Does the parameter type of the setter match the return type of the getter?
at org.springframework.beans.BeanWrapperImpl.createNotWritablePropertyException(BeanWrapperImpl.java:243)
at org.springframework.beans.AbstractNestablePropertyAccessor.processLocalProperty(AbstractNestablePropertyAccessor.java:426)
at org.springframework.beans.AbstractNestablePropertyAccessor.setPropertyValue(AbstractNestablePropertyAccessor.java:278)
at org.springframework.beans.AbstractNestablePropertyAccessor.setPropertyValue(AbstractNestablePropertyAccessor.java:266)
at org.springframework.beans.AbstractPropertyAccessor.setPropertyValues(AbstractPropertyAccessor.java:97)
at org.springframework.beans.AbstractPropertyAccessor.setPropertyValues(AbstractPropertyAccessor.java:77)
at org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.applyPropertyValues(AbstractAutowireCapableBeanFactory.java:1739)
... 13 more
```
抓住關鍵報錯信息:
```
Bean property 'sname' is not writable or has an invalid setter method. Does the parameter type of the setter match the return type of the getter?
```
說的就是沒有```setter()```方法導致bean無法創建。因爲我們的依賴注入通過setter方法底層進行Java```映射```創建的,具體的感興趣的查詢相關的資料。
#### 1.2.3.2 通過構造器進行依賴注入
```xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:p="http://www.springframework.org/schema/p"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.3.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.3.xsd">
<!-- this is bean for student by setter -->
<bean id="student1" class="day0610.pojo.Student">
<property name="sname" value="tom"/>
<property name="sno" value="110001"/>
</bean>
<!-- this is bean for student by constructor -->
<bean id="student2" class="day0610.pojo.Student">
<constructor-arg name="sname" value="pitter"/>
<constructor-arg name="sno" value="110002"/>
</bean>
</beans>
```
```java
package day0610.main;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import day0610.pojo.Student;
public class Test {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
Student student1 = (Student)context.getBean("student1");
System.out.println(student1);
Student student2 = (Student)context.getBean("student2");
System.out.println(student2);
}
}
```
輸出結果:
```
Student [sname=tom, sno=110001]
Student [sname=pitter, sno=110002]
```
我們可以看見通過構造器實現依賴注入,就必須有構造方法。
#### 1.2.3.3 通過p命名空間進行依賴注入
```xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:p="http://www.springframework.org/schema/p"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.3.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.3.xsd">
<!-- this is bean for student by setter -->
<bean id="student1" class="day0610.pojo.Student">
<property name="sname" value="tom"/>
<property name="sno" value="110001"/>
</bean>
<!-- this is bean for student by constructor -->
<bean id="student2" class="day0610.pojo.Student">
<constructor-arg name="sname" value="pitter"/>
<constructor-arg name="sno" value="110002"/>
</bean>
<!-- this is bean for student by p -->
<bean id="student3" class="day0610.pojo.Student" p:sname="lucy" p:sno="110003"></bean>
</beans>
```
```java
package day0610.main;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import day0610.pojo.Student;
public class Test {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
Student student1 = (Student)context.getBean("student1");
System.out.println(student1);
Student student2 = (Student)context.getBean("student2");
System.out.println(student2);
Student student3 = (Student)context.getBean("student3");
System.out.println(student3);
}
}
```
輸出結果:
```
Student [sname=tom, sno=110001]
Student [sname=pitter, sno=110002]
Student [sname=lucy, sno=110003]
```
#### 1.2.3.4 其他常見的注入操作(特殊值、引用類型等等)
我們再加一個POJO類,並修改學生POJO對象
```java
package day0610.pojo;
public class Teacher {
private String tname,tno;
public Teacher(String tname, String tno) {
super();
this.tname = tname;
this.tno = tno;
}
public Teacher() {
super();
}
public String getTname() {
return tname;
}
public void setTname(String tname) {
this.tname = tname;
}
public String getTno() {
return tno;
}
public void setTno(String tno) {
this.tno = tno;
}
@Override
public String toString() {
return "Teacher [tname=" + tname + ", tno=" + tno + "]";
}
}
```
```java
package day0610.pojo;
public class Student {
private String sname,sno;
private Teacher teacher;
public Student() {
super();
}
public Student(String sname, String sno, Teacher teacher) {
super();
this.sname = sname;
this.sno = sno;
this.teacher = teacher;
}
public String getSname() {
return sname;
}
public void setSname(String sname) {
this.sname = sname;
}
public String getSno() {
return sno;
}
public void setSno(String sno) {
this.sno = sno;
}
public Teacher getTeacher() {
return teacher;
}
public void setTeacher(Teacher teacher) {
this.teacher = teacher;
}
@Override
public String toString() {
return "Student [sname=" + sname + ", sno=" + sno + ", teacher=" + teacher + "]";
}
}
```
==引用對象類型==
```xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:p="http://www.springframework.org/schema/p"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.3.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.3.xsd">
<!-- 實例化Teacher -->
<bean id="teacher" class="day0610.pojo.Teacher">
<property name="tname" value="jack"></property>
<property name="tno" value="11000001"></property>
</bean>
<!-- 實例化Student -->
<bean id="student" class="day0610.pojo.Student">
<property name="sname" value="lucy"></property>
<property name="sno" value="110021"></property>
<!-- 引用上面的教師實例 -->
<property name="teacher" ref="teacher"></property>
</bean>
</beans>
```
輸出結果:
```
Student [sname=lucy, sno=110021, teacher=Teacher [tname=jack, tno=11000001]]
```
==特殊值處理==
我們在進行依賴注入的時候爲了解決特殊值的注入一般採用特殊值的實體引用和```<![CDATA[ ]]>```標記,如下表所示爲部分特殊值的實體引用。
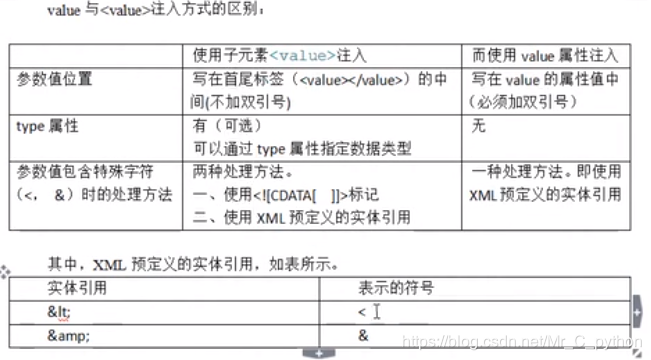
1)帶有```<>```符號
```xml
<!-- 實例化Teacher -->
<bean id="teacher" class="day0610.pojo.Teacher">
<property name="tname">
<value><java課程設計></value>
</property>
<property name="tno" value="11000001"></property>
</bean>
```
2)空值
```xml
<!-- 實例化Teacher -->
<bean id="teacher" class="day0610.pojo.Teacher">
<property name="tname">
<!-- 第一種:啥也不寫就是代表空值 -->
<value></value>
</property>
<property name="tno" value="11000001"></property>
</bean>
```
```xml
<!-- 實例化Teacher -->
<bean id="teacher" class="day0610.pojo.Teacher">
<property name="tname">
<!-- 第二種:使用null標籤 -->
<null></null>
</property>
<property name="tno" value="11000001"></property>
</bean>
```
3)使用```<![CDATA[]]>```包含特殊值
```xml
<!-- 實例化Teacher -->
<bean id="teacher" class="day0610.pojo.Teacher">
<property name="tname">
<value>
<![CDATA[<]]> Java
</value>
</property>
<property name="tno" value="11000001"></property>
</bean>
```
==map類型注入==
```xml
<bean id="teacher" class="day0610.pojo.Teacher">
<property name="tname">
<map>
<entry key="" value=""></entry>
<entry key="" value=""></entry>
<entry key="" value=""></entry>
</map>
</property>
<property name="tno" value="11000001"></property>
</bean>
```
### 1.2.4 自動裝配