一、介紹
JUnit是一款優秀的開源Java單元測試框架,也是目前使用率最高最流行的測試框架,開發工具Eclipse和IDEA對JUnit都有很好的支持,JUnit主要用於白盒測試和迴歸測試。
- 白盒測試:把測試對象看作一個打開的盒子,程序內部的邏輯結構和其他信息對測試人
員是公開的;
- 迴歸測試:軟件或環境修復或更正後的再測試;
- 單元測試:最小粒度的測試,以測試某個功能或代碼塊。一般由程序員來做,因爲它需要知道內部程序設計和編碼的細節;
JUnit GitHub地址:https://github.com/junit-team
# 二、JUnit使用
開發環境:
- Spring Boot 2.0.4 RELEASE
- JUnit 4.12
- Maven
- IDEA 2018.2
## 2.1 檢測JUnit依賴
如果是Spring Boot項目默認已經加入了JUnit框架支持,可在pom.xml中查看:
```xml
org.springframework.boot
spring-boot-starter-test
test
```
如果Maven項目中沒有添加JUnit依賴,可參照如上代碼,手動添加。
## 2.2 基礎使用
簡單的測試代碼如下:
```java
@RunWith(SpringRunner.class)
@SpringBootTest
public class SimpleTest {
@Test
public void doTest() {
int num = new Integer(1);
Assert.assertEquals(num, 1);
}
}
```
在測試類中郵件運行項目,效果如下:
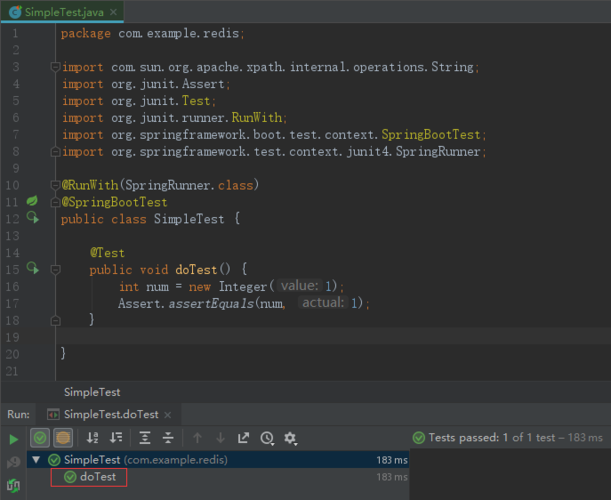
從控制檯可以看出測試通過了。
## 2.3 註解說明
### 2.3.1 註解列表
- @RunWith:標識爲JUnit的運行環境;
- @SpringBootTest:獲取啓動類、加載配置,確定裝載Spring Boot;
- @Test:聲明需要測試的方法;
- @BeforeClass:針對所有測試,只執行一次,且必須爲static void;
- @AfterClass:針對所有測試,只執行一次,且必須爲static void;
- @Before:每個測試方法前都會執行的方法;
- @After:每個測試方法前都會執行的方法;
- @Ignore:忽略方法;
### 2.3.2 超時測試
代碼如下,給Test設置timeout屬性即可,時間單位爲毫秒:
> @Test(timeout = 1000)
## 2.4 斷言測試
斷言測試也就是期望值測試,是單元測試的核心也就是決定測試結果的表達式,Assert對象中的斷言方法:
- Assert.assertEquals 對比兩個值相等
- Assert.assertNotEquals 對比兩個值不相等
- Assert.assertSame 對比兩個對象的引用相等
- Assert.assertArrayEquals 對比兩個數組相等
- Assert.assertTrue 驗證返回是否爲真
- Assert.assertFlase 驗證返回是否爲假
- Assert.assertNull 驗證null
- Assert.assertNotNull 驗證非null
代碼示例如下:
```java
@Test
public void doTest() {
String[] string1 = {"1", "2"};
String[] string2 = string1;
String[] string3 = {"1", "2"};
Assert.assertEquals(string1, string2);
Assert.assertEquals(string2, string3);
Assert.assertSame(string1, string2);
Assert.assertSame(string2, string3); //驗證不通過,string2、string3指向的引用不同
}
```
## 2.5 Web模擬測試
在Spring Boot項目裏面可以直接使用JUnit對web項目進行測試,Spring 提供了“TestRestTemplate”對象,使用這個對象可以很方便的進行模擬請求。
Web測試只需要進行兩步操作:
1. 在@SpringBootTest註解上設置“ebEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT”隨機端口;
2. 使用TestRestTemplate進行post或get請求;
示例代碼如下:
```java
@RunWith(SpringRunner.class)
@SpringBootTest(webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT)
public class UserControllerTest {
@Autowired
private TestRestTemplate restTemplate;
@Test
public void getName() {
String name = restTemplate.getForObject("/name", String.class);
System.out.println(name);
Assert.assertEquals("Adam", name);
}
}
```
其中getForObject的含義代表執行get請求,並返回Object結果,第二個參數設置返回結果爲String類型,更多的請求方法:
- getForEntity:Get請求,返回實體對象(可以是集合);
- postForEntity:Post請求,返回實體對象(可以是集合);
- postForObject:Post請求,返回對象;
## 2.6 數據庫測試
在測試數據操作的時候,我們不想讓測試污染數據庫,也是可以實現的,只需要添加給測試類上添加“@Transactional”即可,這樣既可以測試數據操作方法,又不會污染數據庫了。
示例代碼如下:
```java
@Test
@Transactional
public void saveTest() {
User user = new User();
user.setName("Adam");
user.setAge(19);
user.setPwd("123456");
userRepository.save(user);
System.out.println("userId:" + user.getId());
Assert.assertTrue(user.getId()>0);
}
```
執行效果如下:
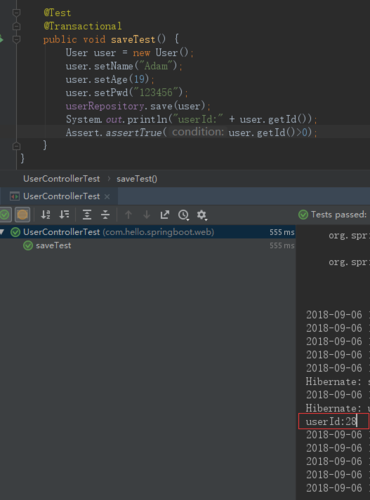
我們可以看到Id有了,也測試通過了,說明數據是添加是正常的,但查看數據庫發現數據裏面是沒有這條數據的。
如果把“@Transactional”去掉的話,數據庫就會正常插入了。
## 2.7 Idea快速開啓測試
在Idea裏面可以快速的添加測試的方法,只需要在要測試的類裏面右鍵選擇“GoTo”點擊“Test”,選擇你需要測試的代碼,點擊生成即可,如果是Windows 用戶可以使用默認快捷鍵“Ctrl + Shift + T”,效果如下圖:
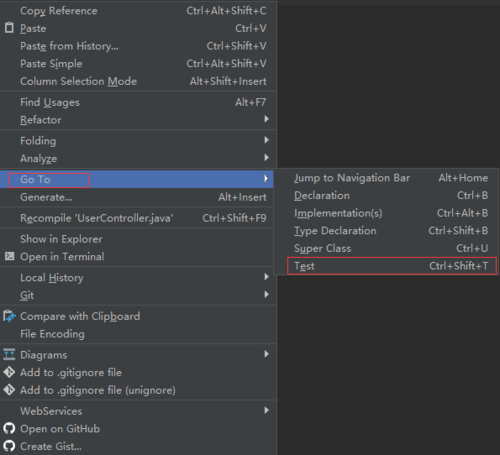
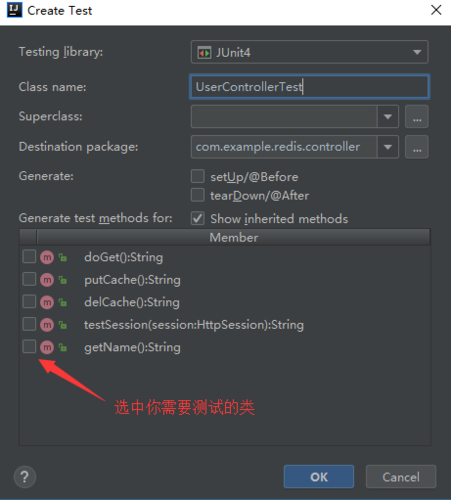
選完方法之後,點擊OK按鈕,就生成了對應的測試代碼,用戶只需要完善框架裏面的具體測試邏輯就可以了。