一、簡介
Redis是一個開源的使用ANSI C語言編寫、支持網絡、可基於內存亦可持久化的日誌型、Key-Value數據庫,並提供多種語言的API,Redis也是技術領域使用最爲廣泛的存儲中間件,它是「Remote Dictionary Service」首字母縮寫,也就是「遠程字典服務」。
Redis相比Memcached提供更多的數據類型支持和數據持久化操作。
# 二、在Docker中安裝Redis
## 2.1 下載鏡像
訪問官網:https://hub.docker.com/r/library/redis/ 選擇下載版本,本文選擇最新Stable 4.0.11
使用命令拉取鏡像:
> docker pull redis:4.0.11
## 2.2 啓動容器
啓動Redis命令如下:
> docker run --name myredis -p 6379:6379 -d redis:4.0.11 redis-server --appendonly yes
命令說明:
- --name 設置別名
- -p 映射宿主端口到容器端口
- -d 後臺運行
- redis-server --appendonly yes 在容器啓動執行redis-server啓動命令,打開redis持久化
啓動成功之後使用命令:
> docker ps
查看redis運行請求,如下圖爲運行成功:

## 2.3 使用客戶端連接
連接Redis不錯的GUI工具應該是Redis Desktop Manager了,不過現在只有Linux版可以免費下載,我上傳了一個Windows版本在百度雲,版本號爲:0.9.5(發佈於2018.08.24)也是比較新的,鏈接: https://pan.baidu.com/s/16npZtnGa3-p2PAafiPEAkA 密碼: 9uqg,還是免安裝的,很好用。
Redis Desktop Manager客戶端預覽:
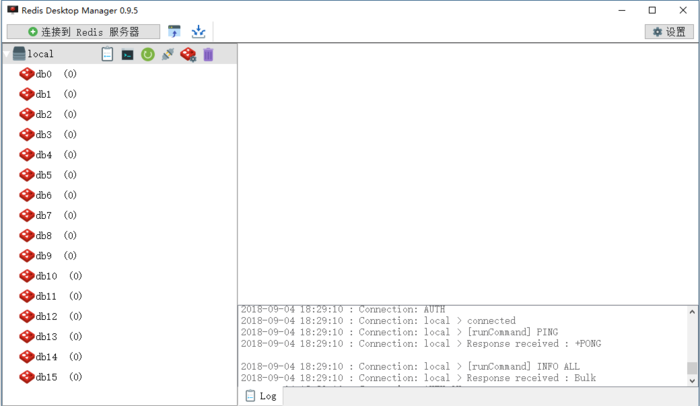
# 三、Redis集成
開發環境
- Spring Boot 2.0.4 RELEASE
- Manven
## 3.1 添加依賴
在pom.xml添加如下依賴:
```xml
org.springframework.boot
spring-boot-starter-data-redis
```
注意不要依賴“spring-boot-starter-redis”它是舊版本,新版已經遷移到“spring-boot-starter-data-redis”了。
## 3.2 配置Redis
在application.properties進行如下設置:
```java
# Redis 配置
# Redis服務器地址
spring.redis.host=127.0.0.1
# Redis服務器連接密碼(默認爲空)
spring.redis.password=
# Redis服務器連接端口
spring.redis.port=6379
# Redis分片(默認爲0)Redis默認有16個分片
spring.redis.database=0
# 連接池最大連接數(使用負值表示沒有限制)
spring.redis.pool.max-active=8
# 連接池最大阻塞等待時間(使用負值表示沒有限制)
spring.redis.pool.max-wait=-1
# 連接池中的最大空閒連接
spring.redis.pool.max-idle=8
# 連接池中的最小空閒連接
spring.redis.pool.min-idle=0
# 連接超時時間(毫秒)
spring.redis.timeout=10000
# 指定spring的緩存爲redis
spring.cache.type=redis
```
注意:spring.redis.timeout不要設置爲0,設置爲0查詢Redis時會報錯,因爲查詢連接時間太短了。
## 3.3 Redis使用
完成以上配置之後就可以寫代碼操作Redis了,示例代碼如下:
```java
@Autowired
private StringRedisTemplate stringRedisTemplate;
@RequestMapping("/")
public String doTest() {
String _key = "time"; //緩存key
stringRedisTemplate.opsForValue().set(_key, String.valueOf(new Date().getTime())); //redis存值
return stringRedisTemplate.opsForValue().get(_key); //redis取值
}
```
更多操作:
- stringRedisTemplate.opsForValue().set("test", "100",60*10,TimeUnit.SECONDS); 向redis裏存入數據和設置緩存時間;
- stringRedisTemplate.hasKey("keyName"); 檢查key是否存在,返回boolean;
# 四、聲明式緩存
爲了簡化緩存可以直接使用聲名式緩存,可以省去設置緩存和讀取緩存的代碼,使用起來會方便很多。
聲明式緩存使用步驟如下:
## 4.1 設置Redis緩存
在pom.xml文件設置緩存爲Redis,代碼如下:
> spring.cache.type=redis
## 4.2 開啓全局緩存
在啓動文件Application.java設置開啓緩存,代碼如下:
```java
@SpringBootApplication
@EnableCaching
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
```
## 4.3 使用註解
註解如下:
- @Cacheable 設置並讀取緩存(第一次設置以後直接讀取);
- @CachePut 更新緩存(每次刪除並更新緩存結果);
- @CacheEvict 刪除緩存(只刪除緩存);
通用屬性:
- value 緩存名稱;
- key 使用SpEL表達式自定義的緩存Key,比如:#name是以參數name爲key的緩存,#resule.name是以返回結果的name作爲key的緩存;
### 4.3.1 @Cacheable 使用
示例代碼如下:
```java
// 緩存key
private final String _CacheKey = "userCacheKeyTime";
@RequestMapping("/")
@Cacheable(value = _CacheKey)
public String index() {
System.out.println("set cache");
return "cache:" + new Date().getTime();
}
```
只有首次訪問的時候會在控制檯打印“set cache”信息,之後直接返回Redis結果了,不會在有添加的打印信息出現。
### 4.3.2 @CachePut 使用
示例代碼如下:
```java
// 緩存key
private final String _CacheKey = "userCacheKeyTime";
@RequestMapping("/put")
@CachePut(value = _CacheKey)
public String putCache() {
System.out.println("update cache");
return "update cache:" + new Date().getTime();
}
```
訪問http://xxx/put 每次會把最新的數據存儲緩存起來。
### 4.3.3 @CacheEvict 使用
示例代碼如下:
```java
// 緩存key
private final String _CacheKey = "userCacheKeyTime";
@RequestMapping("/del")
@CacheEvict(value = _CacheKey)
public String delCache() {
System.out.println("緩存刪除");
return "delete cache:" + new Date().getTime();
}
```
訪問http://xxx/del 只會刪除緩存,除此之後不會進行任何操作。
# 五、分佈式Session共享
在分佈式系統中Session共享有很多種方案,而把Session託管在緩存中是最常用的方案之一,下面來看Session在Redis中的託管步驟。
## 5.1 添加依賴
在pom.xml中添加如下引用:
```xml
org.springframework.session
spring-session-data-redis
```
## 5.2 開啓Session功能
在啓動類Application.java的類註解添加開啓Session,代碼如下:
```java
@SpringBootApplication
@EnableCaching
@EnableRedisHttpSession(maxInactiveIntervalInSeconds = 1800)
public class RedisApplication {
public static void main(String[] args) {
SpringApplication.run(RedisApplication.class, args);
}
}
```
其中maxInactiveIntervalInSeconds爲Session過期時間,默認30分鐘,設置單位爲秒。
## 5.3 Session使用
接下來編寫一段代碼來測試一下Session,示例代碼如下:
```java
@RequestMapping("/uid")
public String testSession(HttpSession session) {
UUID uid = (UUID) session.getAttribute("uid");
if (uid == null) {
uid = UUID.randomUUID();
}
session.setAttribute("uid", uid);
return session.getId();
}
```
連續訪問兩次請求之後,查看控制檯信息如下圖:
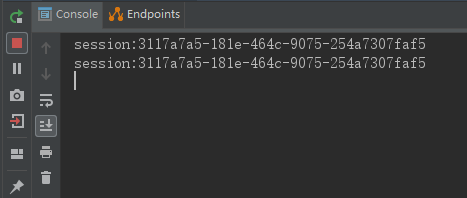
可以看出,兩次訪問的SessionId是一樣的,這個時候在查看Redis 客戶端,如下圖:
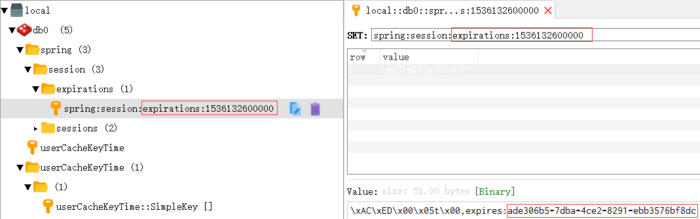
發現Redis裏存儲的Session過期時間也是對的,符合我們的設置。
## 5.4 分佈式系統共享Session
因爲把Session託管給同一臺Redis服務器了,所以Session在Spring Boot中按照如上方式在配置多臺服務器,得到的Session是一樣的。
示例×××:https://github.com/vipstone/springboot-example/tree/master/springboot-redis
**參考資料**
Spring boot中Redis的使用:http://www.ityouknow.com/springboot/2016/03/06/spring-boot-redis.html